Other Efficiency Issues
This is a script file to illustrate other "tricks" to improve execution such as preallocation and smart coding
Note: Preallocate large vectors or matrices
As our example we create a Brownian path
randn('state',20) % initialize the random number generator n = 10000; % set the number of steps
How long does a for-loop take?
tic; dt = 1/n; w(1) = 0; for i=1:n w(i+1) = w(i) + sqrt(dt)*randn; end t1 = toc; plot(0:dt:1,w) fprintf('Time with for-loop: %f seconds\n\n', t1)
Time with for-loop: 0.106239 seconds
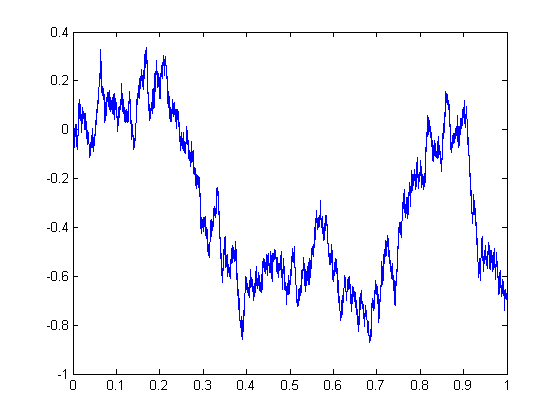
Now we preallocate the vector and try again
clear w tic; dt = 1/n; w = zeros(n+1,1); for i=1:n w(i+1) = w(i) + sqrt(dt)*randn; end t1 = toc; fprintf('Time with preallocation and for-loop: %f seconds\n\n', t1)
Time with preallocation and for-loop: 0.122389 seconds
Here we preallocate the vector and precompute
clear w tic; dt = 1/n; w = zeros(n+1,1); sdt = sqrt(dt); for i=1:n w(i+1) = w(i) + sdt*randn; end t1 = toc; fprintf('Time with preallocation and smart for-loop: %f seconds\n\n', t1)
Time with preallocation and smart for-loop: 0.121054 seconds
Finally, we vectorize the for-loop with cumsum
clear w tic; dt = 1/n; w = sqrt(dt)*cumsum([0;randn(n,1)]); t1 = toc; fprintf('Time with vectorization: %f seconds\n\n', t1)
Time with vectorization: 0.000239 seconds